Security Best Practices
Essential security guidelines and best practices for implementing authentication securely
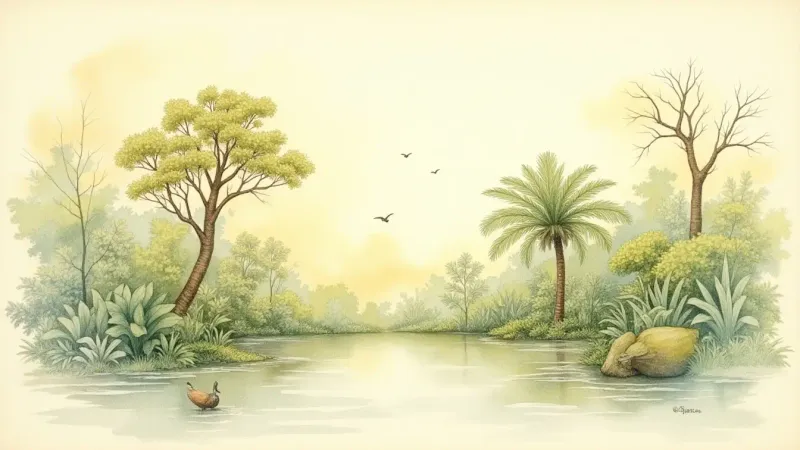
Follow these security best practices to ensure your authentication implementation is secure.
Token Storage
- Never store tokens in localStorage
- Use httpOnly cookies when possible
- Implement secure token refresh flows
PKCE Implementation
Always use PKCE for public clients:
const codeVerifier = generateRandomString();
const codeChallenge = await sha256(codeVerifier);
auth.loginWithRedirect({
code_challenge: codeChallenge,
code_challenge_method: 'S256'
});
API Security
- Always use HTTPS
- Validate tokens on every request
- Implement rate limiting
XSS Prevention
// Sanitize user input
const sanitized = DOMPurify.sanitize(userInput);
CSRF Protection
- Use SameSite cookies
- Implement CSRF tokens
- Validate Origin headers
Regular security audits and penetration testing are recommended.