Rate Limiting Configuration
Protect your authentication endpoints from abuse with configurable rate limiting
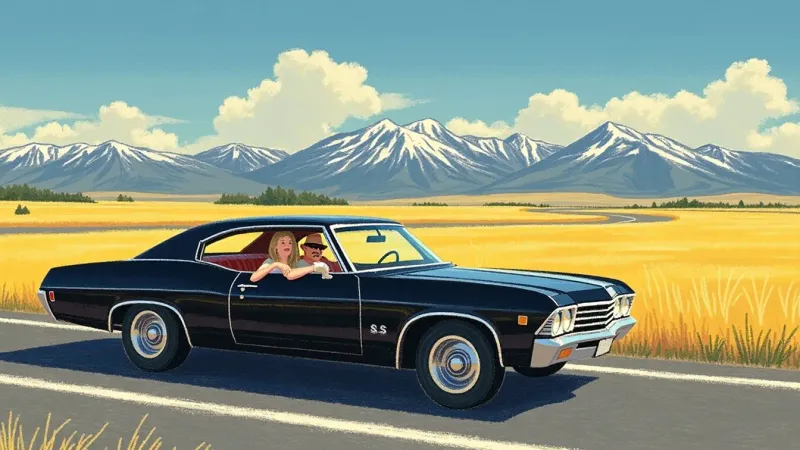
Protect your authentication endpoints with configurable rate limiting rules.
Basic Configuration
rateLimits:
login:
windowMs: 900000 # 15 minutes
max: 5 # limit each IP to 5 requests per windowMs
register:
windowMs: 3600000 # 1 hour
max: 3
passwordReset:
windowMs: 3600000
max: 3
Custom Rules
const customLimiter = rateLimit({
keyGenerator: (req) => {
return req.headers['x-api-key'] || req.ip;
},
handler: (req, res) => {
res.status(429).json({
error: 'Too many requests',
retryAfter: 60
});
}
});
Redis-Based Rate Limiting
const redisStore = new RedisStore({
client: redisClient,
prefix: 'rl:'
});
const limiter = rateLimit({
store: redisStore,
windowMs: 15 * 60 * 1000,
max: 100
});
Bypass Rules
const bypassList = ['trusted-ip-1', 'trusted-ip-2'];
if (bypassList.includes(req.ip)) {
return next();
}
For distributed rate limiting, see our scaling guide.